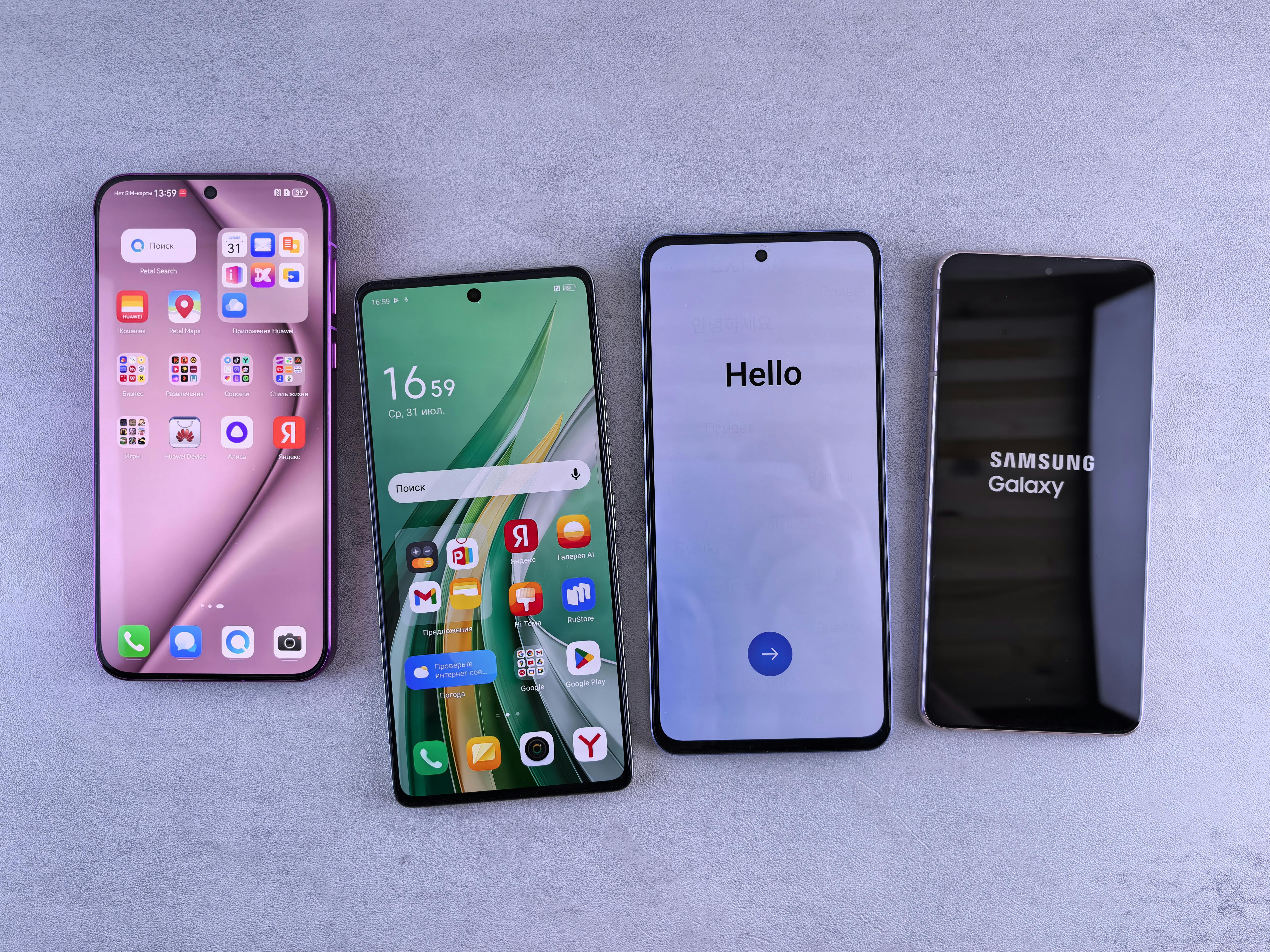
Cross-platform mobile development has evolved dramatically in recent years, with React Native and Flutter emerging as the dominant frameworks. Both promise the coveted "write once, run anywhere" experience, but they take fundamentally different approaches to achieving this goal. This in-depth analysis examines their architectures, performance characteristics, and development experiences to help you make an informed choice for your next project.
Framework Foundations: Understanding the Core Architecture
To properly evaluate these frameworks, we need to understand how they fundamentally differ in their approach to rendering UI components and bridging to native capabilities.
React Native: JavaScript Bridge Architecture
React Native operates on a bridge-based architecture where JavaScript code communicates with native components through a serialization/deserialization process:
- JavaScript code runs in a separate thread (JavaScript Core)
- UI updates require crossing the bridge to native components
- Leverages native platform UI components for rendering
- The new architecture (Fabric and TurboModules) aims to reduce bridge overhead
// React Native Component Example
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const MyComponent = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello from React Native</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
padding: 16,
backgroundColor: '#f0f0f0',
},
text: {
fontSize: 18,
color: '#333',
},
});
export default MyComponent;
Flutter: Direct Rendering Engine
Flutter takes a fundamentally different approach with its own rendering engine called Skia:
- Dart code compiles to native machine code
- Controls every pixel on the screen through its rendering engine
- Does not use platform UI components (draws its own widgets)
- Communicates with the platform through message passing
// Flutter Component Example
import 'package:flutter/material.dart';
class MyComponent extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
padding: EdgeInsets.all(16.0),
color: Color(0xFFf0f0f0),
child: Text(
'Hello from Flutter',
style: TextStyle(
fontSize: 18.0,
color: Color(0xFF333333),
),
),
);
}
}
Performance Comparison: Beyond the Benchmarks
Performance is multi-faceted in mobile development. Rather than simply declaring a winner, let's examine different performance aspects that matter in real-world applications:
Performance Aspect | React Native | Flutter | Why It Matters |
---|---|---|---|
Startup Time | Good | Excellent | First impressions matter; slow startup frustrates users |
Animation Smoothness | Good (requires optimization) | Excellent | Crucial for engaging user experiences |
Memory Usage | Moderate | Low-Moderate | Impacts device compatibility and background performance |
Complex UI Rendering | Good | Excellent | Determines how sophisticated your UI can be |
App Size | Smaller | Larger (but improving) | Affects download conversion rates |
Hot Reload Speed | Fast | Very Fast | Accelerates development iterations |
Real-World Performance Insights
In our experience building production apps with both frameworks:
- Flutter excels at animations, custom UI, and consistent performance across devices
- React Native performs better when integrating deeply with native platform features and for apps needing minimal footprint
- The performance gap has narrowed with React Native's new architecture and Flutter's optimization efforts
- Both frameworks can achieve excellent performance when used appropriately and optimized correctly
React Native Ecosystem
React Native benefits from the mature React ecosystem and JavaScript's widespread adoption.
Key Advantages
- Vast npm ecosystem with over 1.3 million packages
- Mature state management options (Redux, MobX, Recoil, Zustand)
- Easier recruitment due to JavaScript's popularity
- Web developers can transition more easily
- Meta's backing ensures continued development
Notable Limitations
- Inconsistent third-party libraries - quality varies greatly
- Bridge performance bottlenecks (being addressed by new architecture)
- Platform-specific bugs can be challenging to debug
- Dependent on native modules for many device capabilities
Companies Using React Native:
Flutter Ecosystem
Flutter's ecosystem is younger but growing rapidly, with strong backing from Google.
Key Advantages
- Comprehensive widget library out of the box
- Strong tooling with excellent debugging experience
- Predictable performance across different devices
- Single UI codebase with minimal platform-specific code
- Google's backing and use in their products
Notable Limitations
- Smaller package ecosystem (though growing rapidly)
- Steeper learning curve for developers new to Dart
- Larger app size (though improving with app size optimization)
- Less mature web support compared to React Native Web
Companies Using Flutter:
Case Studies: Framework Selection in Practice
Case 1: E-commerce App with Heavy Animation
Project Requirements:
- Rich product browsing experience
- Complex animations and transitions
- Custom UI design system
- Consistent performance on low-end devices
Framework Choice: Flutter
Flutter was selected for its superior animation performance and pixel-perfect control over UI elements, ensuring a consistent shopping experience across diverse Android devices.
Case 2: Enterprise Productivity App
Project Requirements:
- Integration with existing JavaScript codebase
- Deep platform-specific integrations
- Extensive form handling
- Team already familiar with React
Framework Choice: React Native
React Native was chosen for its seamless integration with existing web components and to leverage the team's React expertise. Platform-specific modules were used to access native APIs where needed.
Making Your Decision: Framework Selection Guide
The "right" framework depends entirely on your specific project needs and constraints. Consider these factors when making your selection:
Choose React Native when:
- Your team has strong JavaScript/React experience
- You need to share code with a React web app
- Deep integration with native APIs is required
- Minimizing app size is critical
- You need access to a wide variety of third-party libraries
Choose Flutter when:
- Custom UI and animations are central to your app
- You need predictable performance across devices
- Your team is willing to learn Dart
- You value a comprehensive, integrated toolkit
- You want to target mobile, web, and desktop from a single codebase
Consider both when:
- Building a prototype to test both approaches
- You have a mixed-skill development team
- Your project has diverse technical requirements
- You're starting a new project without legacy constraints
- Long-term maintainability is a key concern
"The best tool isn't the one with the most features or best performance in benchmarks—it's the one that best fits your specific project needs, team skills, and future direction."
The Future Landscape
Both frameworks continue to evolve rapidly. Here's what to watch for in the near future:
React Native's Evolution
- The New Architecture (Fabric renderer and TurboModules) will significantly improve performance
- React Server Components may bring new patterns to mobile development
- Stronger web integration through React Native Web improvements
- Enhanced TypeScript integration for better type safety
Flutter's Roadmap
- Flutter 3.0 improvements focused on performance and web rendering
- Ongoing Impeller renderer development for iOS and future platforms
- Advanced tooling with DevTools enhancements
- Material You and design system improvements
Conclusion: Beyond the Framework
While the choice between React Native and Flutter is important, remember that successful mobile apps depend on factors beyond the framework:
User-Centered Design
Focus on solving real user problems with intuitive interfaces, regardless of framework.
Quality Architecture
A well-structured codebase with proper separation of concerns will outlive any framework.
Performance Optimization
Both frameworks can deliver excellent performance when developers understand and optimize for their strengths.
The most successful mobile apps focus on delivering value to users through thoughtful design, robust architecture, and performance optimization—principles that transcend the specific framework choice.